Java
- Details
- Written by: scelac
- Category: Java
- Hits: 143
Database testing has always been a challenging aspect of software development. When it comes to testing the JDBC layer, developers often face a dilemma: use mocks (which don't test actual database interactions) or use a real database (which can be slow and difficult to set up consistently). This is where TestContainers comes in as a game-changer for your Java database testing strategy.
Read more: Unit Testing the JDBC Layer Using TestContainers and Liquibase
- Details
- Written by: scelac
- Category: Java
- Hits: 718
Checking if a string contains at least one capital letter is a common requirement in Java programming. This is especially relevant in scenarios like validating passwords, formatting user inputs, or implementing security checks. In Java 21, there are several efficient ways to achieve this, each with its own advantages.
Read more: How to check if a String has at least one capital letter in Java
- Details
- Written by: scelac
- Category: Java
- Hits: 152
Java is a powerful programming language known for its performance optimizations and memory management techniques. One such optimization is the Integer caching mechanism, which plays a significant role in improving efficiency when dealing with frequently used integer values. This blog post will explore the concept of Integer caching, how it works in Java, and how developers can leverage or override this feature to optimize their applications.
- Details
- Written by: scelac
- Category: Java
- Hits: 230
The SOLID design principles are essential for writing clean, structured, and maintainable code. A strong grasp of these principles is a crucial skill for any programmer, as they form the foundation upon which many design patterns are built. These principles, introduced by Robert C. Martin (Uncle Bob), are widely used in object-oriented programming (OOP) to write clean and manageable code.
- Details
- Written by: scelac
- Category: Java
- Hits: 129
Transaction handling in JDBC (Java Database Connectivity) is a crucial aspect of database management that ensures data integrity and consistency. When working with multiple SQL operations, transactions allow developers to commit or rollback changes based on execution success or failure.
- Details
- Written by: scelac
- Category: Java
- Hits: 235
Unit testing the JDBC (Java Database Connectivity) layer is crucial for building robust and maintainable database-driven applications. Many developers struggle with testing JDBC code because it directly interacts with external databases. However, with the right approach, you can effectively unit test your JDBC components, ensuring they function as expected.
- Details
- Written by: scelac
- Category: Java
- Hits: 126
Programming paradigms define how we approach software development, influencing how we structure and reason about our code. Among them, functional programming (FP) has gained increasing popularity due to its emphasis on pure functions, immutability, and declarative programming. Unlike imperative or object-oriented programming, FP encourages writing stateless and side-effect-free code, leading to more predictable and maintainable applications.
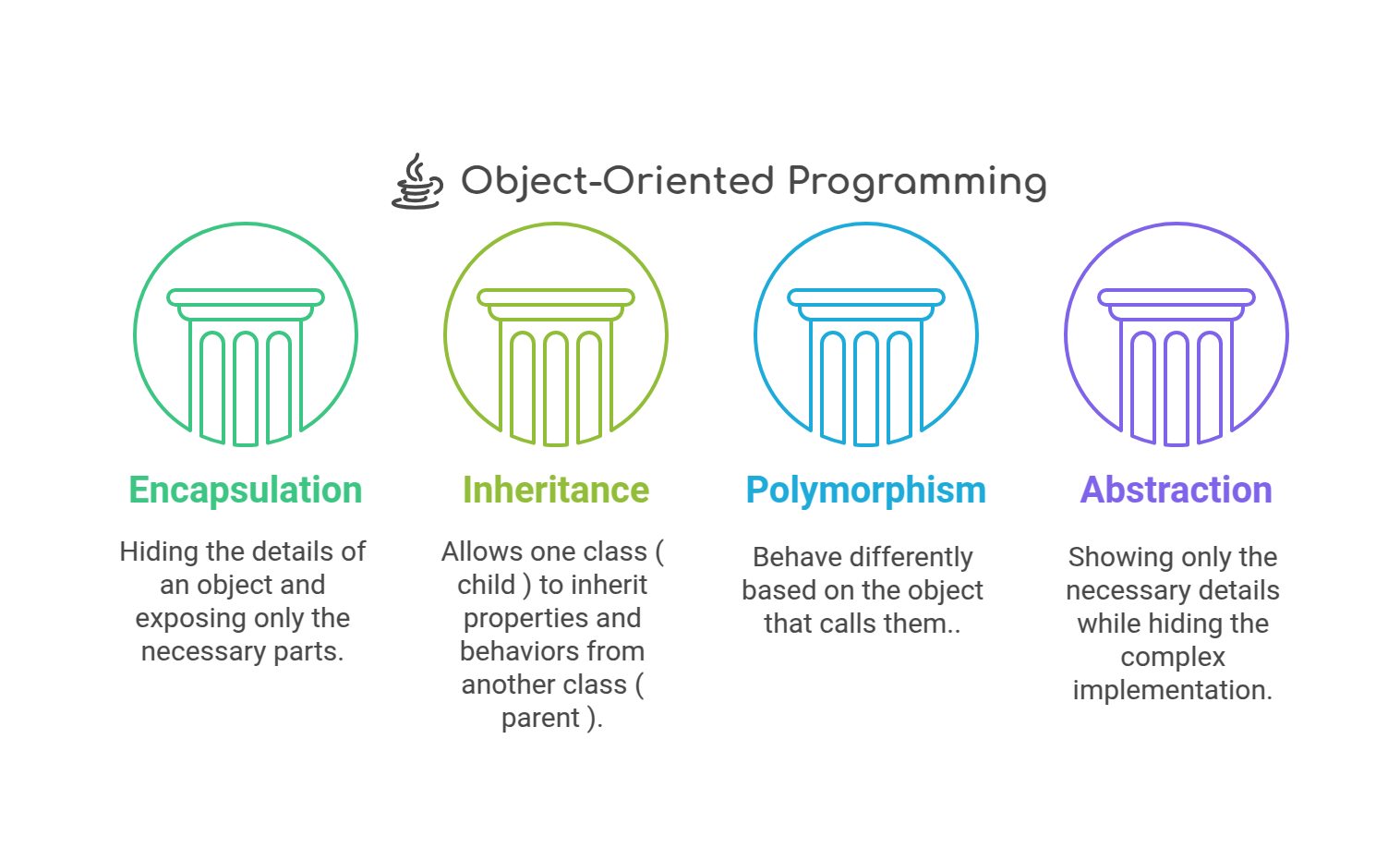
- Details
- Written by: scelac
- Category: Java
- Hits: 288
If you're diving into Java, you've probably heard the term Object-Oriented Programming (OOP) thrown around a lot. But what exactly is OOP, and why is it such a big deal in Java? Don’t worry—I got you. This article is for your, no-jargon guide to understanding OOP in Java, contains real-world examples, best practices, and a few fun analogies to make things stick.
Read more: Understanding Object-Oriented Programming (OOP) in Java
- Details
- Written by: scelac
- Category: Java
- Hits: 152
Database connection pooling is essential for optimizing application performance by efficiently managing database connections. HikariCP, known for its speed and lightweight footprint, is a preferred choice for Java applications using JDBC. This guide will show you how to implement HikariCP for efficient connection pooling in your Java project.