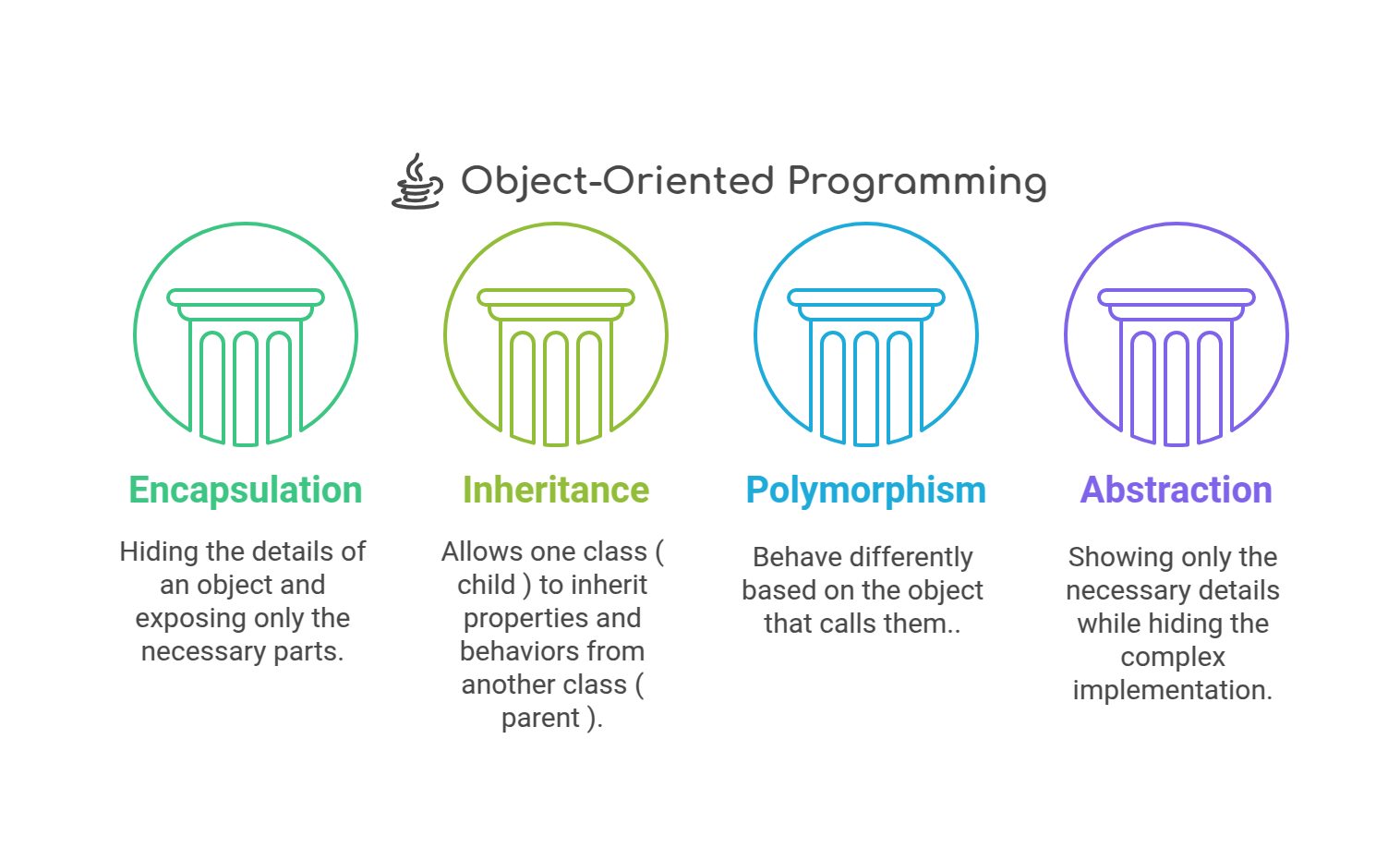
Understanding Object-Oriented Programming (OOP) in Java
- Details
- Category: Java
- Hits: 124
If you're diving into Java, you've probably heard the term Object-Oriented Programming (OOP) thrown around a lot. But what exactly is OOP, and why is it such a big deal in Java? Don’t worry—I got you. This article is for your, no-jargon guide to understanding OOP in Java, contains real-world examples, best practices, and a few fun analogies to make things stick.
Read more: Understanding Object-Oriented Programming (OOP) in Java
Unit testing of the JDBC layer using JUnit 5
- Details
- Category: Java
- Hits: 124
Unit testing the JDBC (Java Database Connectivity) layer is crucial for building robust and maintainable database-driven applications. Many developers struggle with testing JDBC code because it directly interacts with external databases. However, with the right approach, you can effectively unit test your JDBC components, ensuring they function as expected.
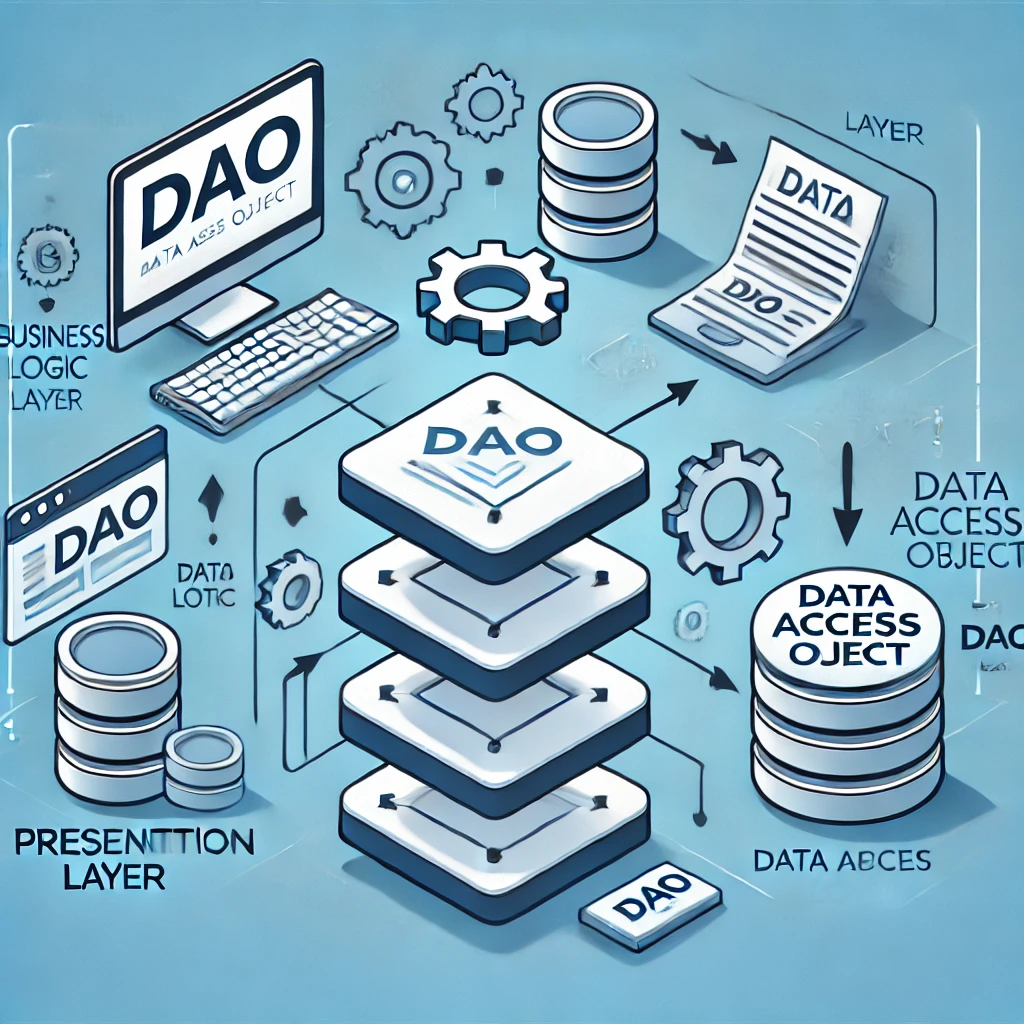
Implement DAO pattern in java
- Details
- Category: Java
- Hits: 177
The Data Access Object (DAO) pattern is a widely used design pattern in Java for managing database interactions in an efficient and structured manner. It separates the persistence logic from the business logic, improving code maintainability, reusability, and scalability. In this article, we will explore the DAO pattern, its advantages, and how to implement it in Java.
SOLID Principles in Java
- Details
- Category: Java
- Hits: 96
The SOLID design principles are essential for writing clean, structured, and maintainable code. A strong grasp of these principles is a crucial skill for any programmer, as they form the foundation upon which many design patterns are built. These principles, introduced by Robert C. Martin (Uncle Bob), are widely used in object-oriented programming (OOP) to write clean and manageable code.
Handling Pagination with JDBC
- Details
- Category: Java
- Hits: 137
When dealing with large datasets in Java applications, retrieving all records at once can be inefficient and resource-intensive. Instead, pagination is used to fetch data in manageable chunks, improving performance and user experience. This blog explores how to implement JDBC pagination effectively, using a single SQL's LIMIT
and OFFSET
clauses also via the implementation of PageRequest
and PageResponse
objects to optimize data retrieval in relational databases.
How to check if a String has at least one capital letter in Java
- Details
- Category: Java
- Hits: 278
Checking if a string contains at least one capital letter is a common requirement in Java programming. This is especially relevant in scenarios like validating passwords, formatting user inputs, or implementing security checks. In Java 21, there are several efficient ways to achieve this, each with its own advantages.
Read more: How to check if a String has at least one capital letter in Java
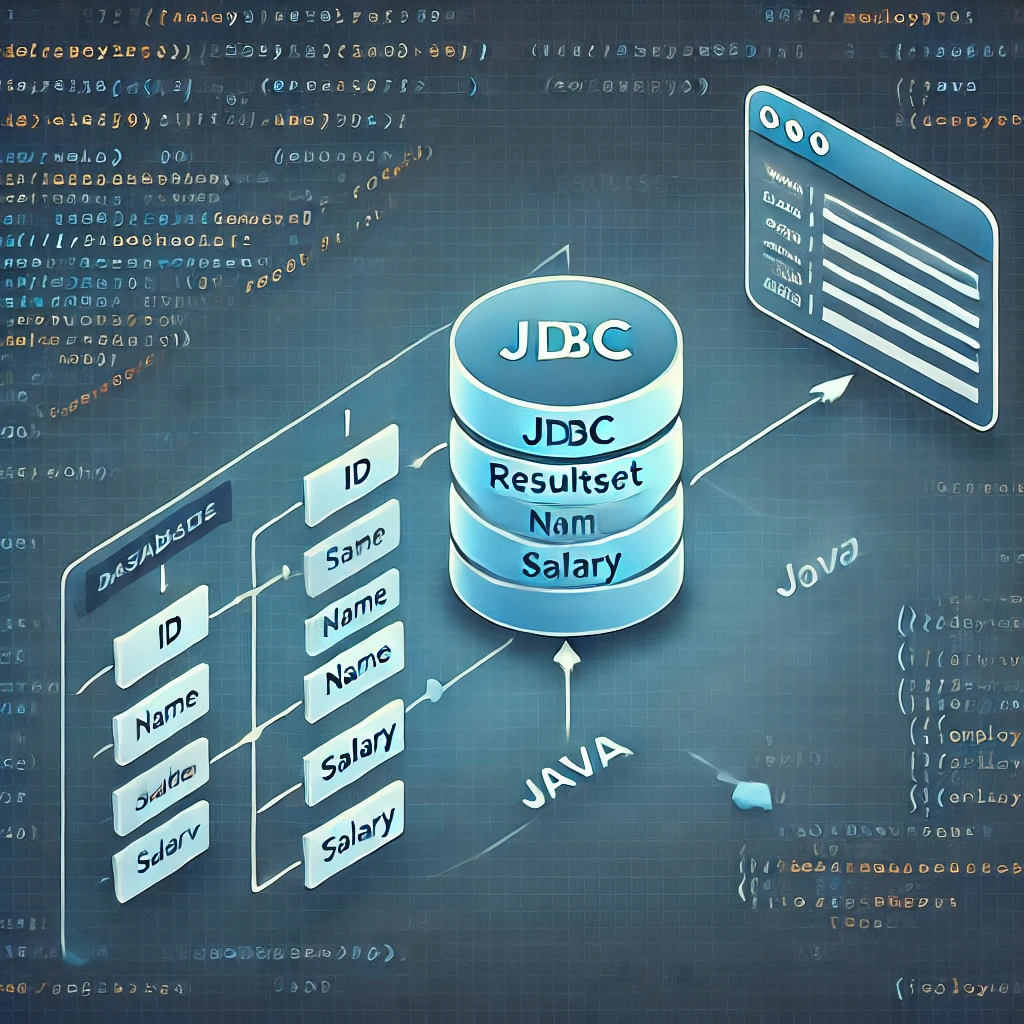
Processing JDBC ResultSet
- Details
- Category: Java
- Hits: 146
JDBC (Java Database Connectivity) is the standard API in Java that connects with relational databases. The ResultSet
is a key component of JDBC, allowing developers to retrieve and process query results. Optimizing the handling of ResultSet
can improve performance and ensure efficient resource management.
Batch Processing in JDBC
- Details
- Category: Java
- Hits: 130
Batch processing is a powerful feature in JDBC (Java Database Connectivity) that allows developers to execute multiple SQL statements efficiently in a single transaction. This technique significantly improves performance when dealing with bulk database operations, such as inserting or updating a large number of records.
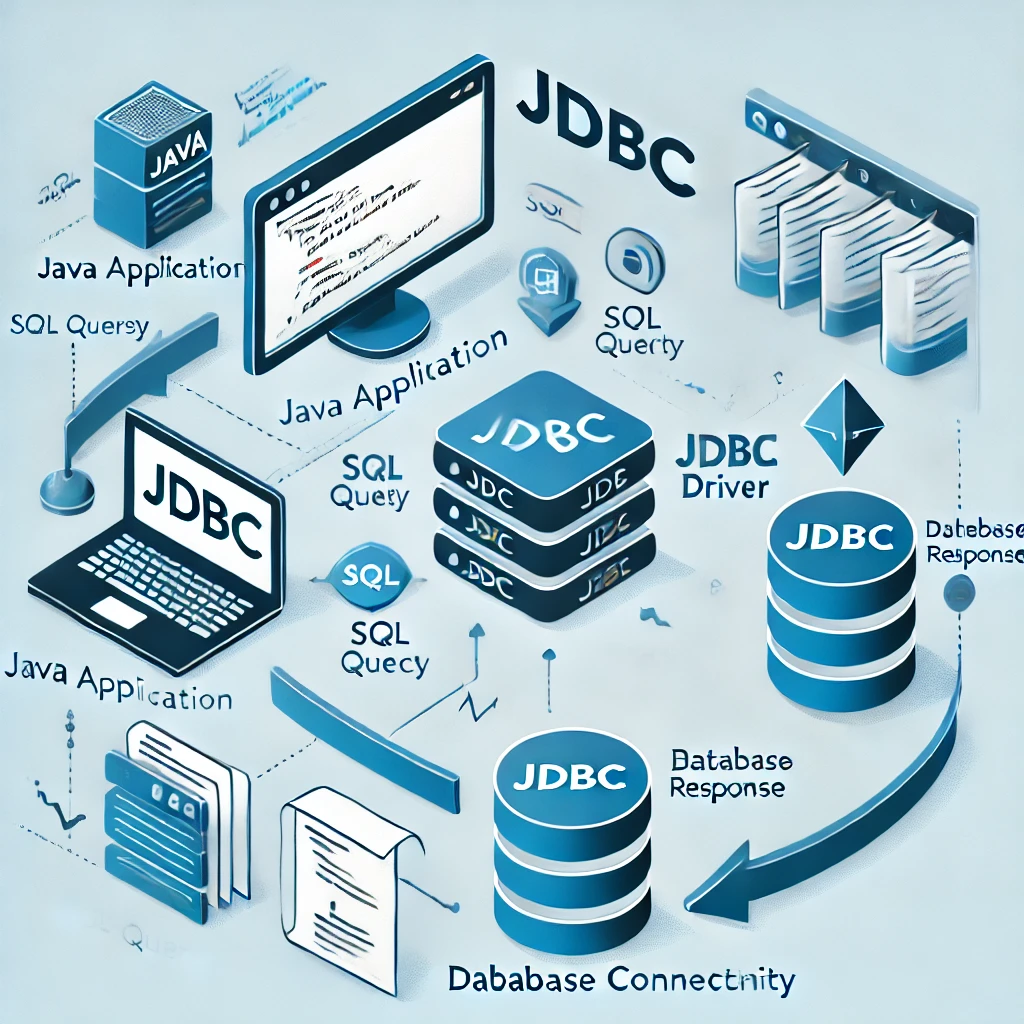
JDBC - Java Database Connectivity
- Details
- Category: Java
- Hits: 173
Java Database Connectivity (JDBC) is an API provided by Java that allows developers to connect and interact with databases. With Java 21, new enhancements in the language and libraries further improve JDBC usage, making database operations more efficient and developer-friendly.
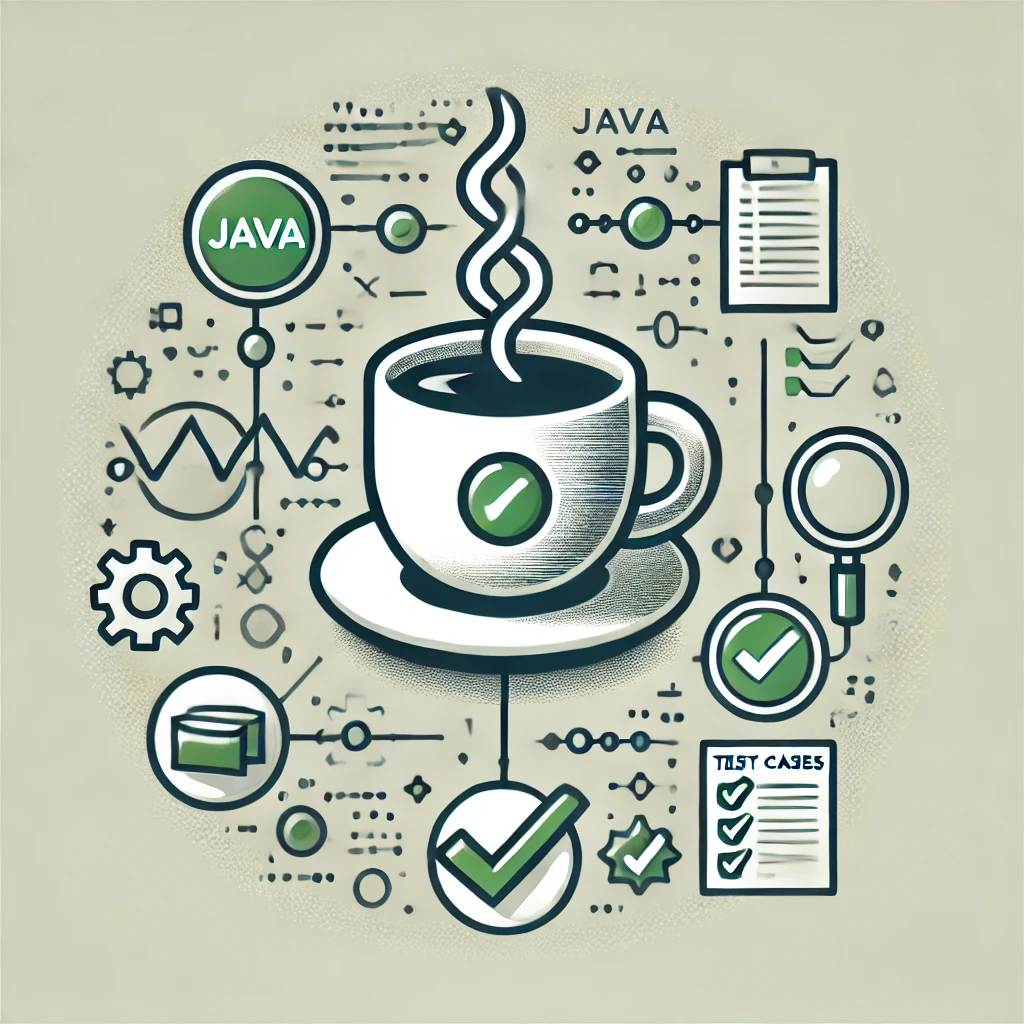
Mockito Framework Tutorial
- Details
- Category: Java
- Hits: 173
Mockito is a powerful Java framework used for unit testing by creating mock objects. It helps developers isolate components of a system, making testing easier, faster, and more reliable. In this tutorial, we'll cover the basics of using Mockito, including creating mocks, stubbing methods, verifying behavior, and more.
Page 1 of 2